Computing π
The Algorithm:
Select random points in the northeast quadrant of unit square and compute the ratio of points in the circle over the total points. Multiply the
result by 4.
Approximately ((Π/4)/1)*4 = Π
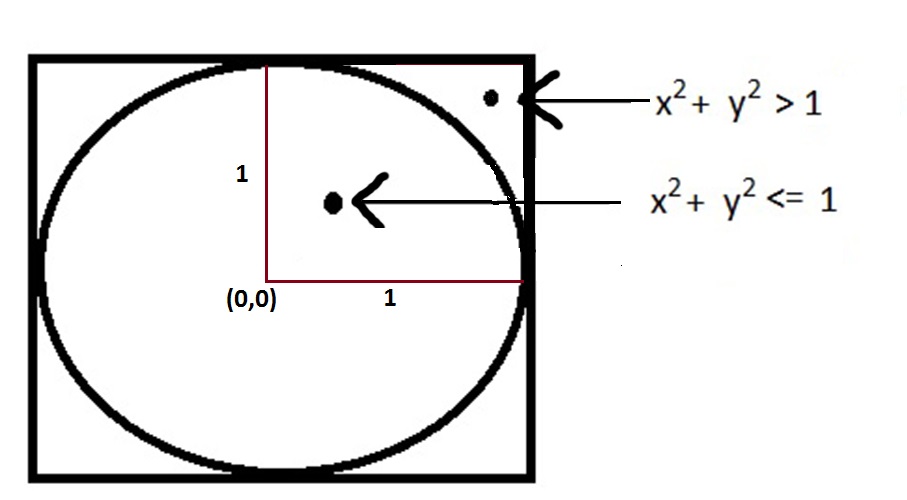
#include <stdlib.h>
#include <stdio.h>
#include <math.h>
#include <string.h>
#include <time.h>
main(int argc, char* argv[])
{
long SEED; // SEED=35791246 Just an example
long n_iterate=0;
double x,y;
int i,count=0; /* # of points in the 1st quadrant of unit circle */
double z;
double pi;
time_t s_seconds,e_seconds;
printf("RAND_MAX= %ld\n",RAND_MAX);
printf("Enter the SEED: ");
scanf("%ld",&SEED);
printf("Enter the number of iterations used to estimate pi: ");
scanf("%ld",&n_iterate);
s_seconds = time(NULL);
/* initialize random numbers */
srand(SEED);
count=0;
for ( i=0; i<n_iterate; i++) {
x = (double)rand()/RAND_MAX;
y = (double)rand()/RAND_MAX;
z = x*x+y*y;
if (z<=1) count++;
}
pi=((double)count/n_iterate)*4;
printf("# of trials= %d , estimate of pi is %g \n",n_iterate,pi);
e_seconds = time(NULL);
printf("Runtime in seconds: %ld\n", (e_seconds-s_seconds));
return 0;
}
Runs
Since each point selection is, for computational purposes, independent we could have processors running the code in parallel and
then average the results. The issue is that "rand()" is determined by SEED (see the image below) so some initial work has to be done,
choosing SEEDs
SEEDing